Pure functions are the building blocks of functional programming. They are adored for simplicity and testability.
In this article you will find a
checklist that will help determine whether a net function or not.
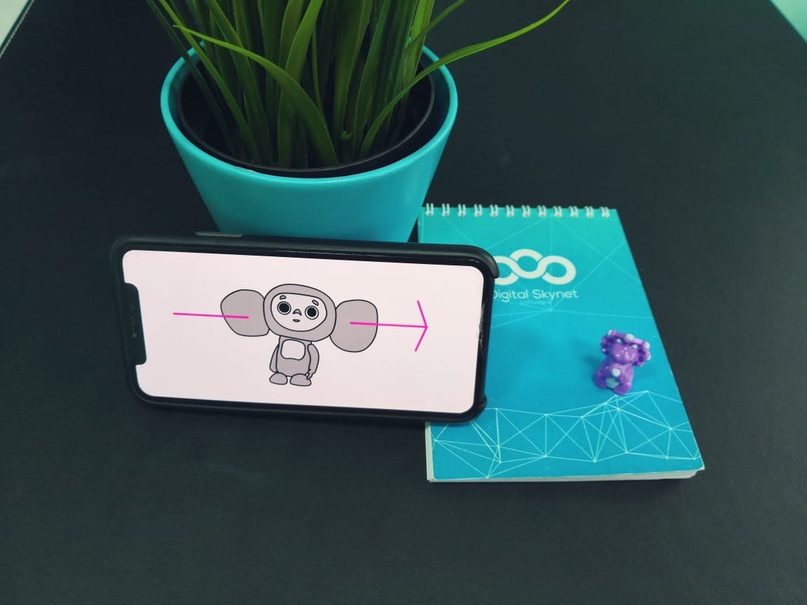
Check list
A function must satisfy two conditions in order to be considered “pure”:- Each time the function returns the same result when it is called with the same set of arguments.
- No side effects
Consider more.
1. Single input => Single output
Compare this to:
const add = (x, y) => x + y; add(2, 4); // 6
With this:
let x = 2; const add = (y) => { x += y; }; add(4); // x === 6 (the first time)
In the first case, the value is returned based on the specified parameters, regardless of where / when you call it.
If you add 2 and 4, always get 6.
Nothing affects the result.
Unclean functions = inconsistent resultsThe second example returns nothing. He relies on the general condition to do his job by increasing the variable outside of his scope.
This model is a nightmare for developers.
The shared state introduces a dependence on time. You get different results depending on when you called the function. The first time result is 6, the next time 10 and so on.
What's better?In which case you get less bugs that appear only under certain conditions?
In which case, are you more likely to succeed in a multi-threaded environment where temporal dependencies can break the system?
Definitely in the first.
2. No side effects
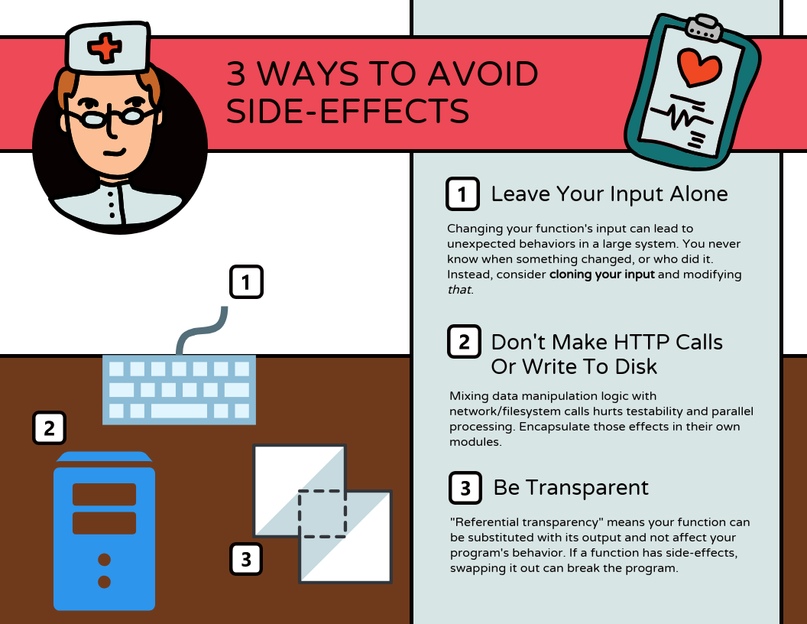
This test is itself a checklist.
Examples of side effects:- Modifying input parameters
- console.log
- HTTP calls (AJAX / fetch)
- File system change
- DOM Requests
In fact, any work performed by a function is not related to the calculation of the final result.
I advise you to watch the
video of Bob Martin.
Here is the “unclean” side effect function.
const impureDouble = (x) => { console.log('doubling', x); return x * 2; }; const result = impureDouble(4); console.log({ result });
console.log here is a side effect, but it doesn't hurt. We still get the same results, given the same data.
However, this can cause problems.
“Unclean” object change
const impureAssoc = (key, value, object) => { object[key] = value; }; const person = { name: 'Bobo' }; const result = impureAssoc('shoeSize', 400, person); console.log({ person, result });
The person variable has been changed forever because the function was declared through an assignment operator.
A shared state means that the effect of
impureAssoc is no longer completely obvious. Understanding the impact on the system now includes tracking every variable it has ever touched and knowing its history.
Shared state = time dependencies.We can clear
impureAssoc by simply returning a new object with the desired properties.
“Clearing it” const pureAssoc = (key, value, object) => ({ ...object, [key]: value }); const person = { name: 'Bobo' }; const result = pureAssoc('shoeSize', 400, person); console.log({ person, result });
Now
pureAssoc returns the test result, and you should not worry if it changes somewhere else.
It was possible to do this:
const pureAssoc = (key, value, object) => { const newObject = { ...object }; newObject[key] = value; return newObject; }; const person = { name: 'Bobo' }; const result = pureAssoc('shoeSize', 400, person); console.log({ person, result });
Changing input data can be dangerous, but changing their copy is not a problem. The end result is a tested, predictable function that works no matter where and when you call it.
Changes are limited to this small area, and you still return value.
Summary- The function is pure if it has no side effects and returns the same result each time when it is called with the same set of arguments.
- Side effects include: changing input, HTTP calls, writing to disk, displaying.
- You can safely clone and then change the input parameters. Just leave the original unchanged.
- The distribution syntax (... syntax) is the easiest way to clone objects and arrays.