Good day.
Today we will talk about the movement of an object by pressing a button on the screen in Unity3D. This theme is very simple, but often used in all sorts of games.
To begin, let's create an empty 3D project. Open Unity, press the button in the upper right corner with the inscription "New". In the field “Project name” we indicate the name of the project. In the line "Location" indicate the location of the project on disk. Below the “Location” field, check the “3D” box and click “Create project”.
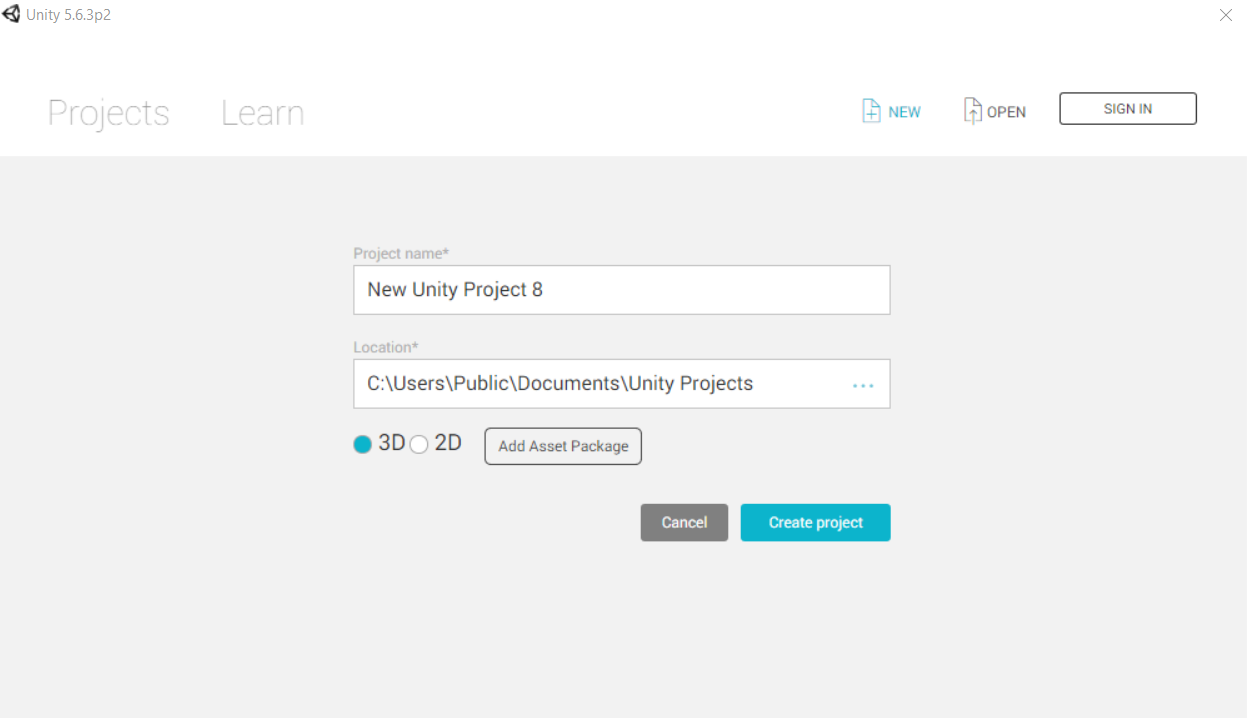
Then we create a cube that will replace our land (GameObject-> 3D Object-> Cube). Then we will make it more flat and stretch it. Open the “Inspector” window, select our cube, in the “Transform” parameter, select the “Scale” item x: 10, y: 0.2, z: 10. We should get this canvas:

Now move the camera to the coordinates x: 0, y: 5, z: 0. Then rotate it 90 degrees along the x axis. Then we need to create an object that we will move. Create a sphere (GameObject-> 3D Object-> Sphere), set the “Position” parameter in the “Transform” as x: 0, y: 1, z: 0. After that, in order to better see our scene, look for an object with the name “Directional Light” on the “Hierarchy” tab and set the “Rotation” parameter in the “Transform” to it as x: 180, y: -30, z: 0. Our project should now look like this:
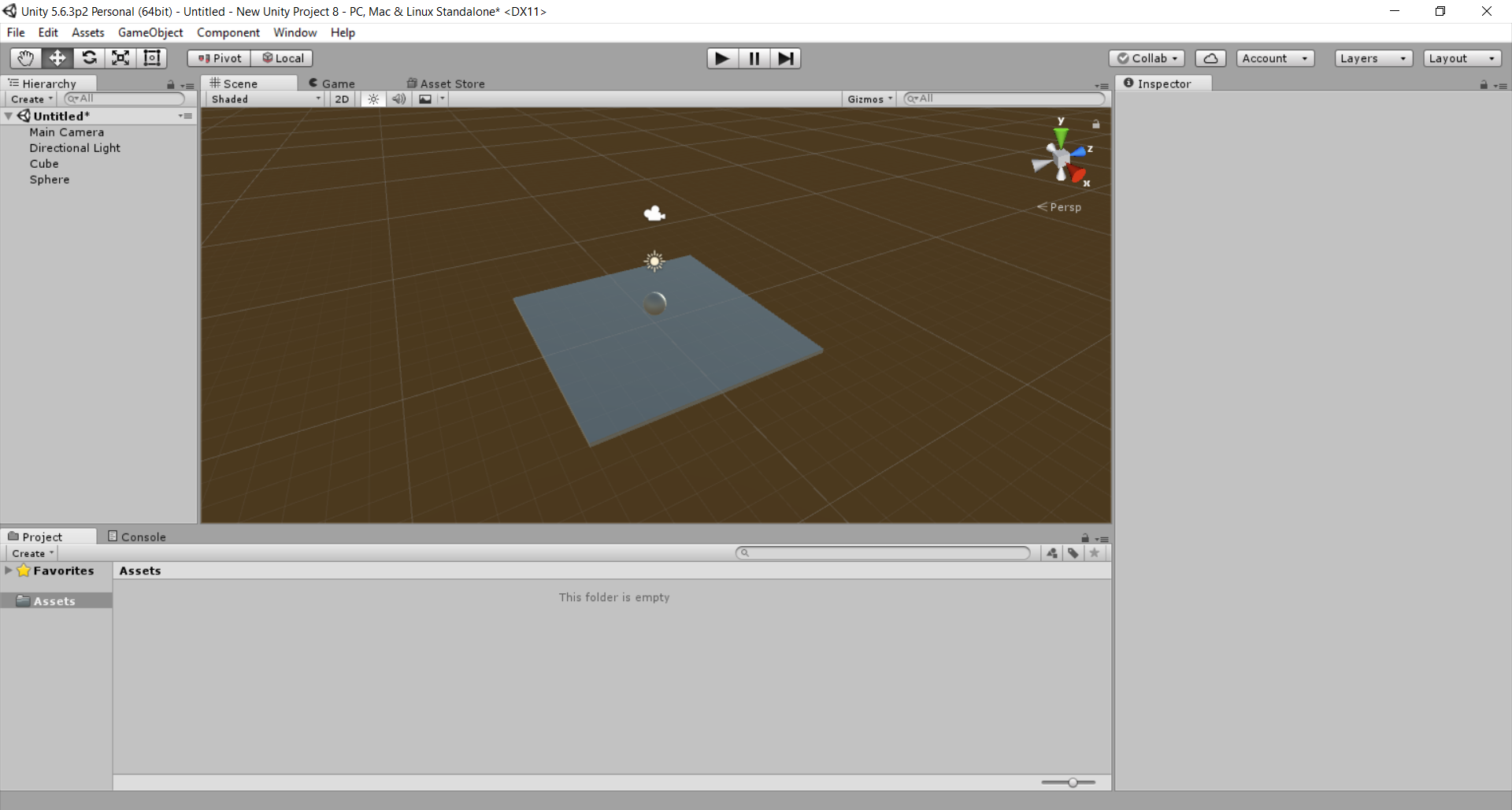
Now we need a button, by pressing which we will determine whether to move our object. First we create a screen on which our button will be located (GameObject-> UI-> Canvas). The next step is to create a button (GameObject-> UI-> Button). Then you need to write a script to move our sphere. In the "Project" window, select "Create", and then select C # Script. The following is the code:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Move : MonoBehaviour {
First create a variable of our object to move. The second variable is the distance to which we will move the object. The public method “OnButtonDown” is responsible for moving when a button is pressed. First, we set the variable S to a value of 1 or 2, after which we move the object along the X axis by S distance.
Then select our button, in the “Inspector” panel at the very bottom, click the “Add Component” button, select our code in “Scripts”. Further, in the “Button” component, in the “On Click ()” item below, press "+". Click on the resulting window in the “On Click ()” parameter in the field where “None (Object)” stands. In it we select our button. Here’s how it should look:

Then in our “On Click ()” parameter we select the block in which “No Function” stands, inside it we select the name of our code, and in it select the “OnButtonDown ()” method. Now, when the button is configured, go to the code. In the "Inspector" window should display the component with the name of our code. In the field "Sphere" choose our sphere. This is what should work:

Then in the “Game” window click on “Maximize On Play”. Now click on the game launch button. Our button is displayed on the screen. If you click on it, then our sphere will move along the X axis.
Before clicking:

After clicking:

Many thanks for reading the publication. I hope you gained new knowledge for yourself.