Good day.
When I first dealt with the composer, I sketched a small cheat sheet for myself and now, after a while, I present it to the public in a slightly modified form.
This publication is relevant to those who first encountered the indispensable package manager for PHP.
So, Composer is a package manager for PHP.
What is Composer and the simplest example of its use for?
Take for example
this project.If in a nutshell: this is a set of scripts for working in the VK API
Accordingly, for the operation of these scripts you need several libraries.
Libraries are listed in the file composer.json - the key file when working with composer
Example composer.json:

This project uses 5 libraries. Accordingly, if the developer decides to publish this project on github, then it is enough for him to throw the folder with scripts into the turnips and composer.json, which will describe the libraries necessary for the work of this project. The simplicity is obvious: the turnips do not need to follow all the necessary libraries following the trailer files. Takes up less space, easier to distribute the project.
Work example:
Download the project
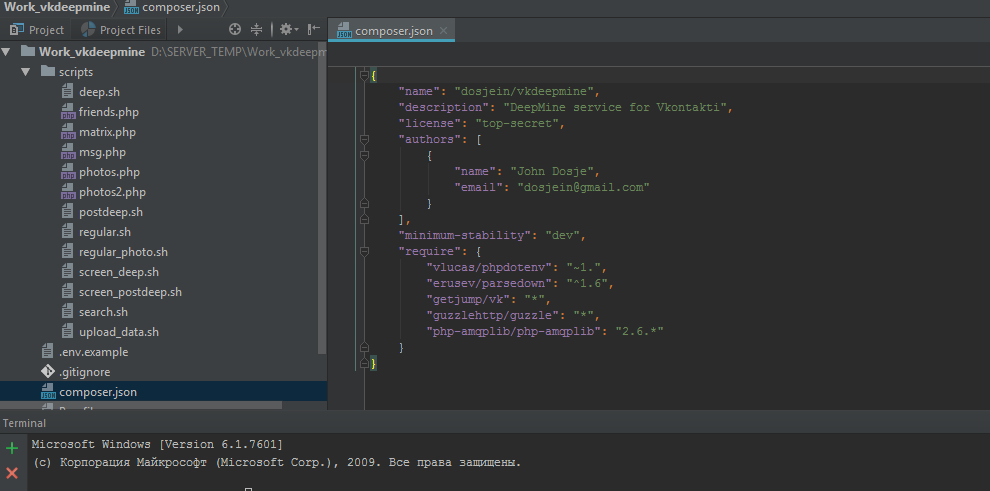
The scripts folder directly contains the project scripts, for which these 5 packages are required.
Run the installation of packages:

After installation, the vendor folder appears, where the installed packages are folded and the file autoload.php is formed

We connect this file to the project and everything - the libraries are connected, you can safely work with them.

The simplicity is obvious: you do not need to download and connect libraries and their dependencies yourself, the composer will do everything for you. And this whole bundle is connected with a single autoload.php file.
All packages that are in the vendor are added to the autoloader. In this case, composer relies on the files composer.json, which should be in each package. Creating a composer.json package is the task of the package developer, all that is required of the package consumer is to describe in the project composer.json which packages need to be included.
This is an example of the composer.json project:
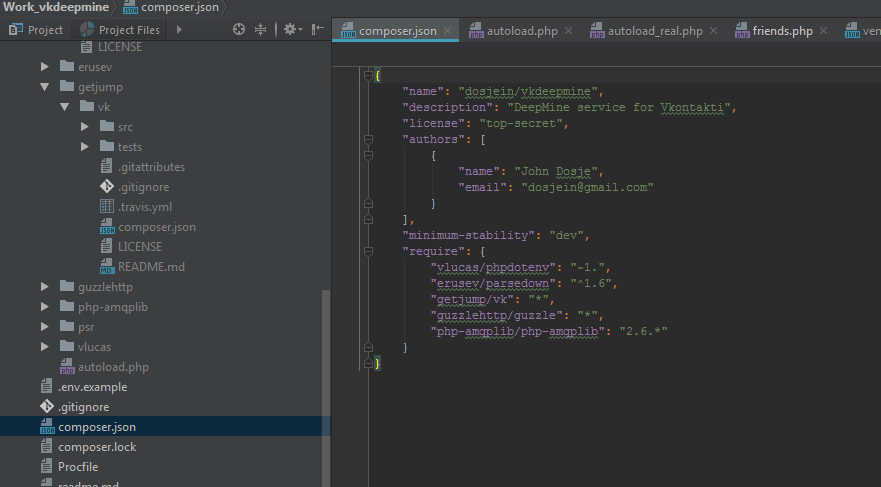
This is an example composer.json package:
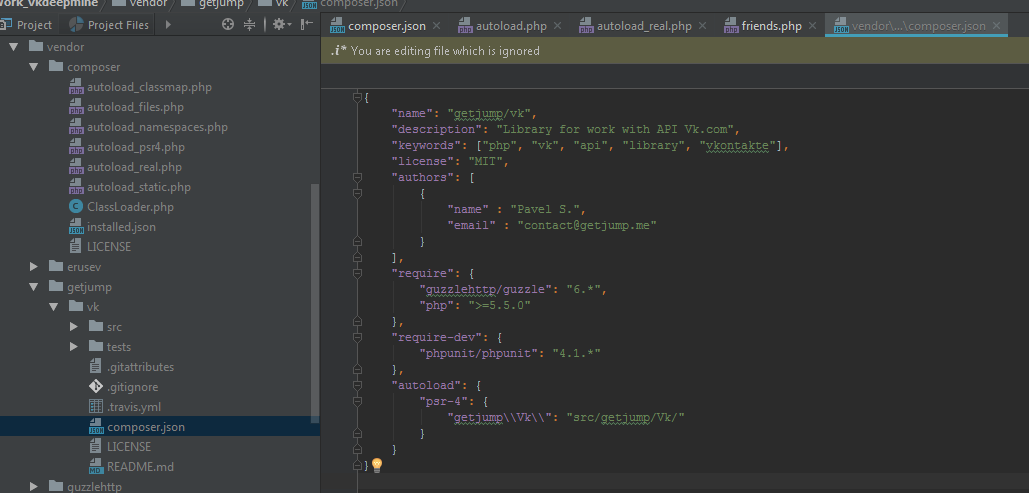
The require section contains a dependency for this package — the guzzle http library, which is required for the getjump / vk library to work. In this case, i.e. from the point of view of the consumer of packages, all sorts of package dependencies are not our “concern”, composer will deal with dependencies itself.
The namespace of the package is written in the autoload section
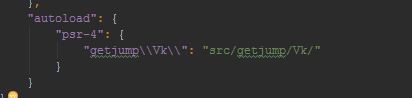
getjump \\ Vk \\ - namespace name
src / getjump / Vk / - directory where files with package classes are located
Working with this library in a project:

Core and Friends are classes of the library, which are laid out and written in the src folder in accordance with the PSR-4 standard. Again, the formation of the package structure is the work of the package creator.
We, as a consumer of the package, are enough to register in our project
include '../vendor/autoload.php';
and all these classes and namespaces will work just fine.
In this case, we do not need to bother and write autoloader. Composer will do this itself when executing the install command.
Installation
Composer installation globally
1) First you need to have the path to the directory with the PHP interpreter be registered in the environment variable path.
Check if this is:
php –version
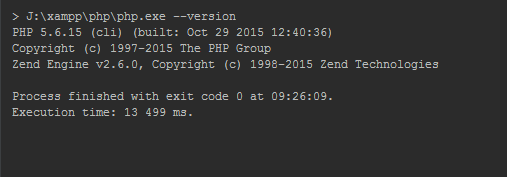
If the output is like this, then you can skip this step.
On the example of Windows 7
System -> Advanced System Settings -> Advanced -> Environment Variables
Next we will be interested in the path variable:

Enter the path to the interpreter

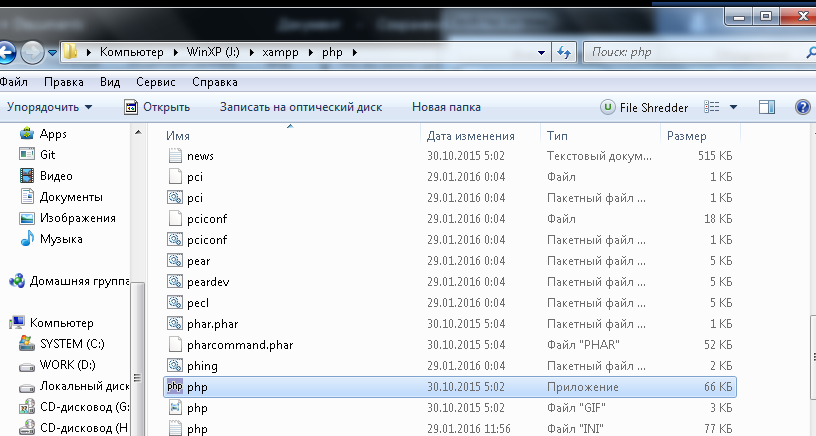
* For a long time, the xampp assembly is on my computer, the assembly itself is not needed here, but the interpreter from it is quite suitable (PHP version - 5.6).
2) Restart the terminal.
Create a directory and set composer (I put it on disk D)
D:
cd /
mkdir bin
cd bin
php -r "readfile ('https://getcomposer.org/installer');" | php
echo
php "% ~ dp0composer.phar"% *> composer.bat
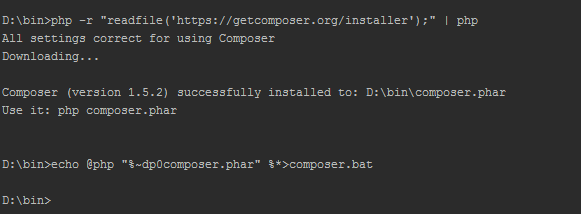
3) Add the path to composer.bat to the environment variable path, for example, for D: \ bin should be:

Additionally, you can add to the path
D: \ Users \% userName% \ AppData \ Roaming \ Composer \ vendor \ bin \
in order to make it easier to use the tools globally installed through Composer.
(I have the Users folder located on disk D, and a C has created a symlink for it).
That's it, the composer is installed and fully operational.
More: you can catch an error during installation
[RuntimeException]
Composer to correctly configure the APPDATA or COMPOSER_HOME environment variable
The solution was found here
github.com/composer/composer/issues/2033Add an APPDATA variable with the value D: \ Users \ GSU \ AppData \ Roaming

Install Composer locally
There is an option to put the composer locally, but in most cases there is no obvious need for this.
However, the installation is even easier.
Since the program is not globally installed, you need a boot file (composer mini-program), to download it we write the command:
php -r "readfile ('https://getcomposer.org/installer');" | php
now the composer.phar file has appeared in the project directory
Everything can be used.
php composer.phar require [package name]
Differences between global and local installation
Commands are run differently with local and global installations:
For example:
Local: php composer.phar require silex / silex ~ 1.1
Global: composer require silex / silex ~ 1.1
For a local installation, you need to download the installation file to the current project folder every time.
php -r "readfile ('https://getcomposer.org/installer');" | php
With global installation, this file is not needed. Composer runs under any current directory.
Teams
install - install packages prescribed in composer.json
update - update packages
dumpautoload - rebuild autoloader
require somepackage / somepackage: someversion - add a new package (by default, packages are installed from the official repository). When installing the package is written in composer.json
update --lock - update the composer.lock lock file
config --global cache-files-maxsize "2048MiB" - an example of changing the configuration parameter
--profile - adding this parameter to any command will enable the display of the execution time and the amount of memory used.
--verbose - detailed information about the operation being performed
show --installed - a list of installed packages with a description of each
show --platform - PHP information
--dry-run - rehearsal command execution. May be added to the install and update commands. Emulates the execution of a command without its direct execution. Required to check if the installation of packages and dependencies will succeed.
remove - remove the package. The exact opposite of require
Syntax composer.json
Package Naming and Package Description Options
The package name consists of two parts separated by a slash: the supplier name (vendor name) and the library name.

If the package is designed in accordance with the PSR-4 standard, but is not published on packagist.org, but on github, then instead of the version of the package, you need to register a branch and repository for this package:

An example of connecting the library, which lies on github, but is not designed according to the PSR-4 standard, but is an ordinary jumble of files with classes and functions.
Approximate view:

Pqr / superlib is the same “wrong” library.
In the repositories section for her we write such a construction.
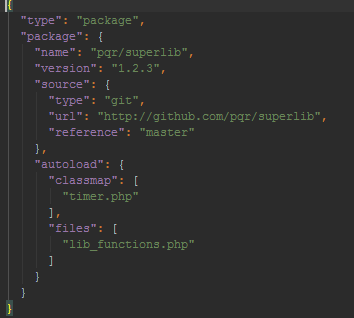
The key point is the autoload section, here we specify the files we need with classes and functions.
Library structure:

File Contents:

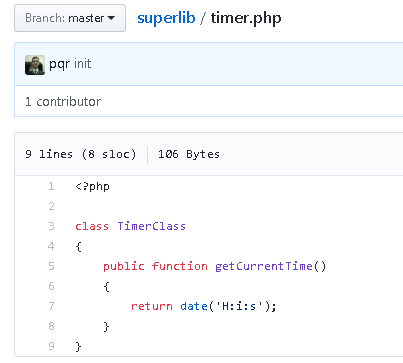
Accordingly, in the project, the call to getCurrentTime () will look something like this:
$ timer = new pqr \ superlib \ TimerClass;
echo $ timer-> getCurrentTime ();
Versioning
When specifying acceptable package versions, you can use exact matching (1.2.3), ranges with comparison operators (<1.2.3), combinations of these operators (> 1.2.3 <1.3), “last available” (1.2. *), Tilde symbol (~ 1.2.3) and the caret (^ 1.2.3).
The tilde specification (~ 1.2.3) will include all versions up to 1.3 (not inclusive), since in semantic versioning this is the moment of introducing new functionality. In this case, the last of the stable minor versions will be obtained. Those. only the last digit will change - 1.2.5, 1.2.8 and so on.
Indicating the insertion mark (^ 1.2.3) literally means “be wary of only critical changes” and will include versions up to 2.0. With regard to semantic versioning, changing the major version is the moment of making critical changes to the project, so versions 1.3, 1.4 and 1.9 are suitable, while 2.0 is no longer.
Those. only the first digit does not change.
Tilde: ~ 1.2.3 is the most common and secure way to specify a version.
Composer.lock file
The composer.lock file saves the current list of installed dependencies and their versions. Thus, at the moment when the dependency versions are already updated (update command), other people who will clone your project will receive the same versions. This allows you to make sure that everyone who receives your project has a batch environment identical to the one you used during development, and helps to avoid errors that could arise from updating versions.
Each time the update command is executed, the updated versions of the packages are written to composer.lock. This file is pushed under the version control system and when installing packages on a new server, those versions of packages that are written in this file will be delivered. When executing the install command, the composer will primarily rely on composer.lock. Thus, on different servers the same batch environment will be guaranteed in terms of versions.
Also, the composer.lock file contains a hash of the composer.json file.
And if the json file has been edited, the composer will warn you that the lock file does not match the json file.
In this case, you need to run the command composer update --lock, which will update composer.lock.
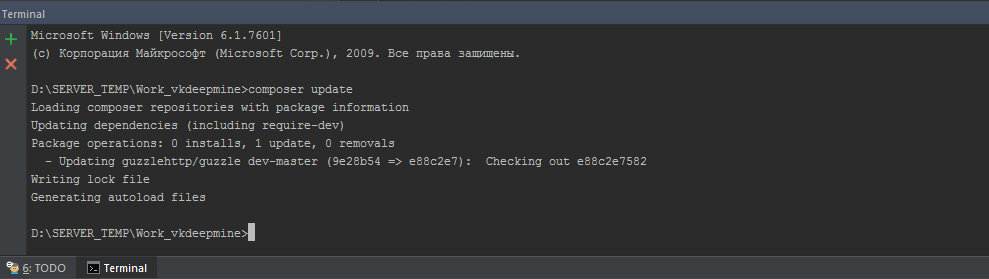
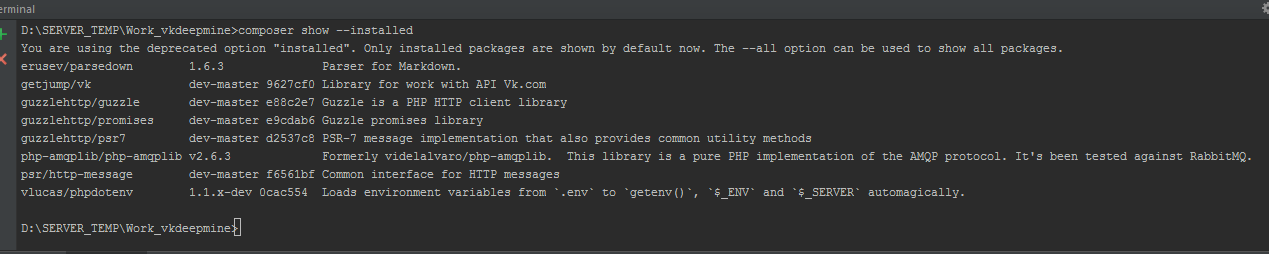
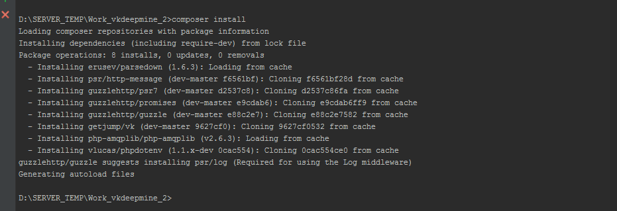
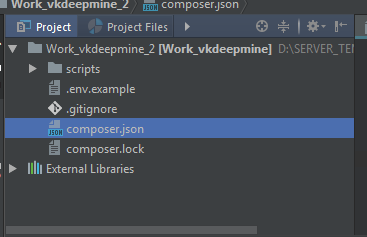
Differences install from update in the context of using composer.lock
The
composer install command does the following:
Checks if composer.lock exists:
- if not, resolves the dependencies and creates it.
- if composer.lock exists, installs the versions specified in it
The
composer update command:
- Checks composer.json
- Determines the latest versions based on those specified in this file.
- Installs the latest versions
- Updates composer.lock in accordance with the established
Example of use from the point of view of the creator of the project
There is a project without installed packages
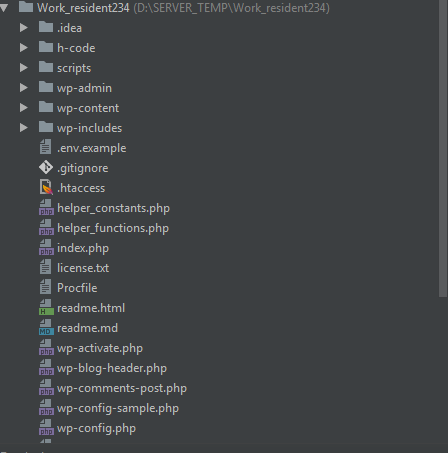
Put several libraries
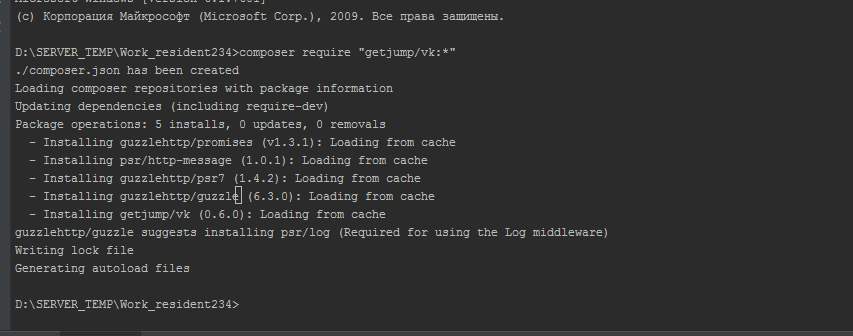

We have composer.json with information about packages.
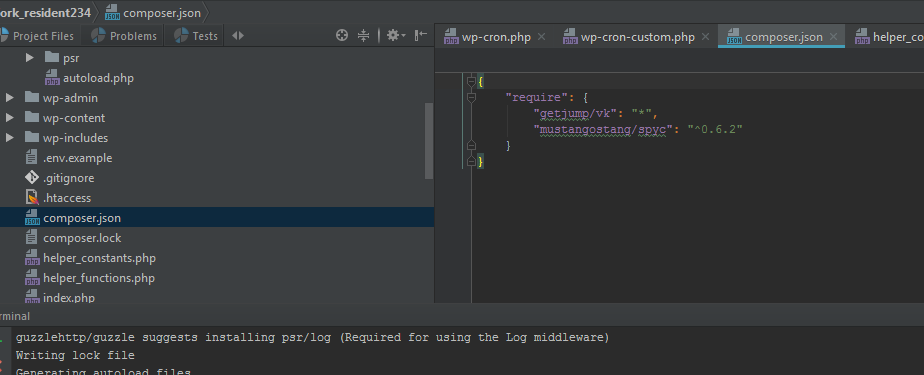
We can add it and distribute the project with this file.
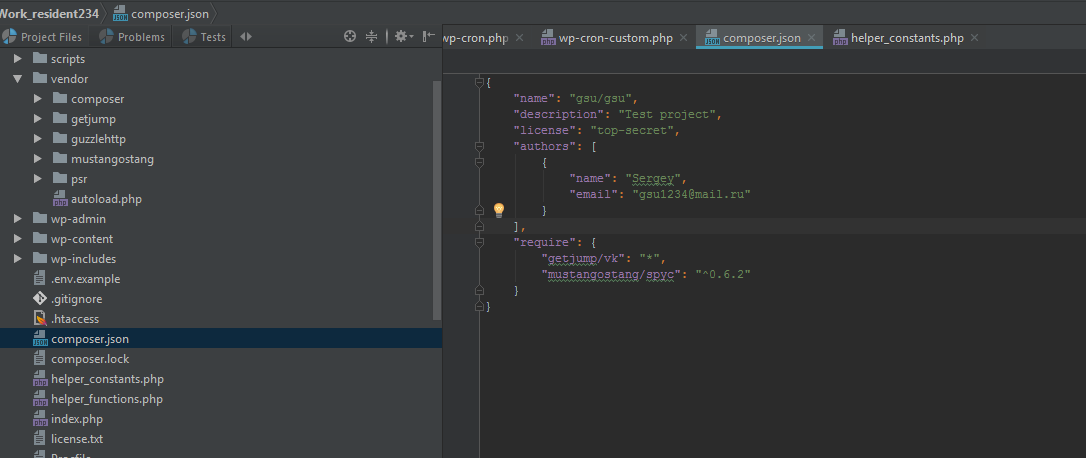
Another user downloaded our project, executed install, and all necessary packages were deployed in his project.
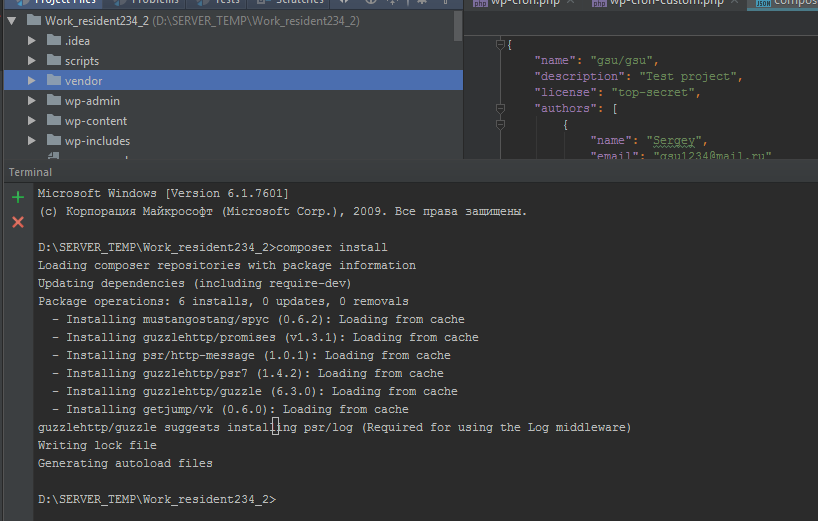
Example of use from the point of view of the package creator
For example, I created a class with a method that will display the URL of the current page.
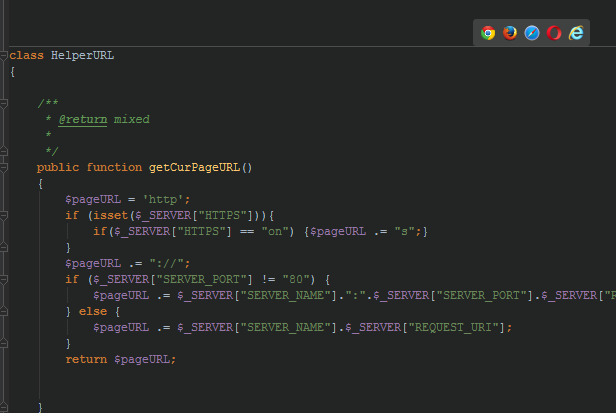
The class is designed as a package and uploaded to github.
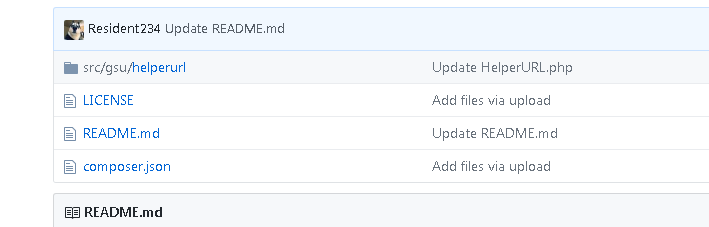
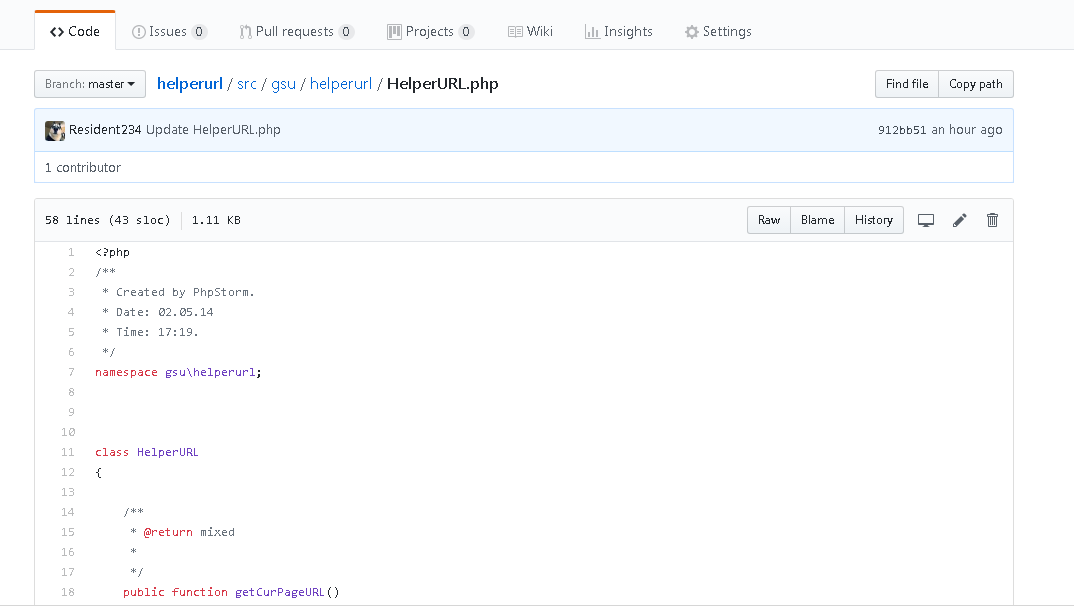
Compiled by composer.json

Register at the office. repositories and add the package, indicating a link to the repository, in which it lies
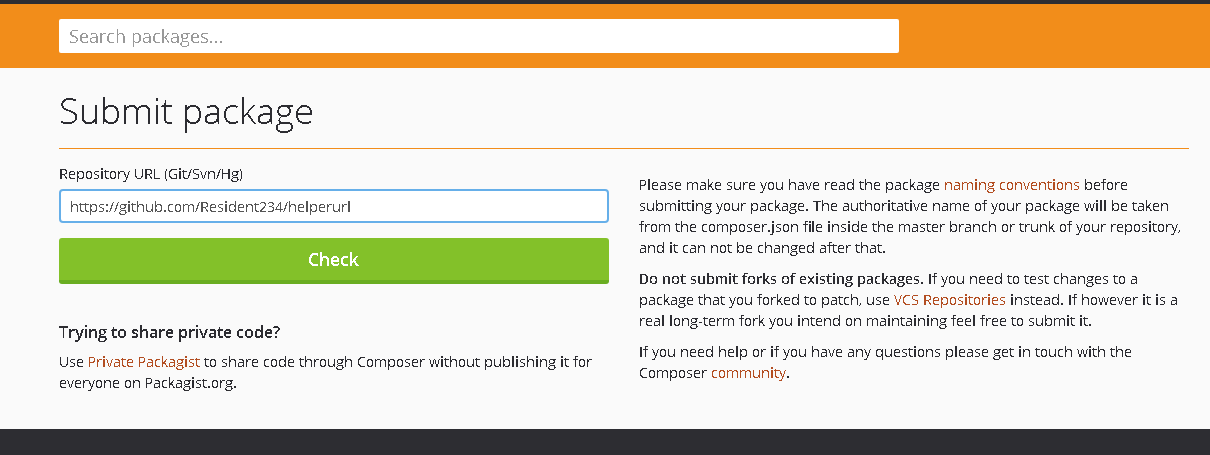
All package added
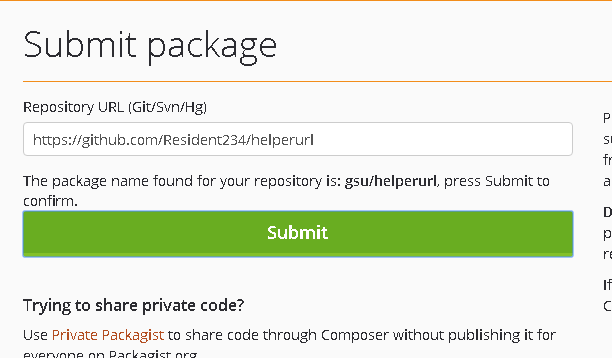
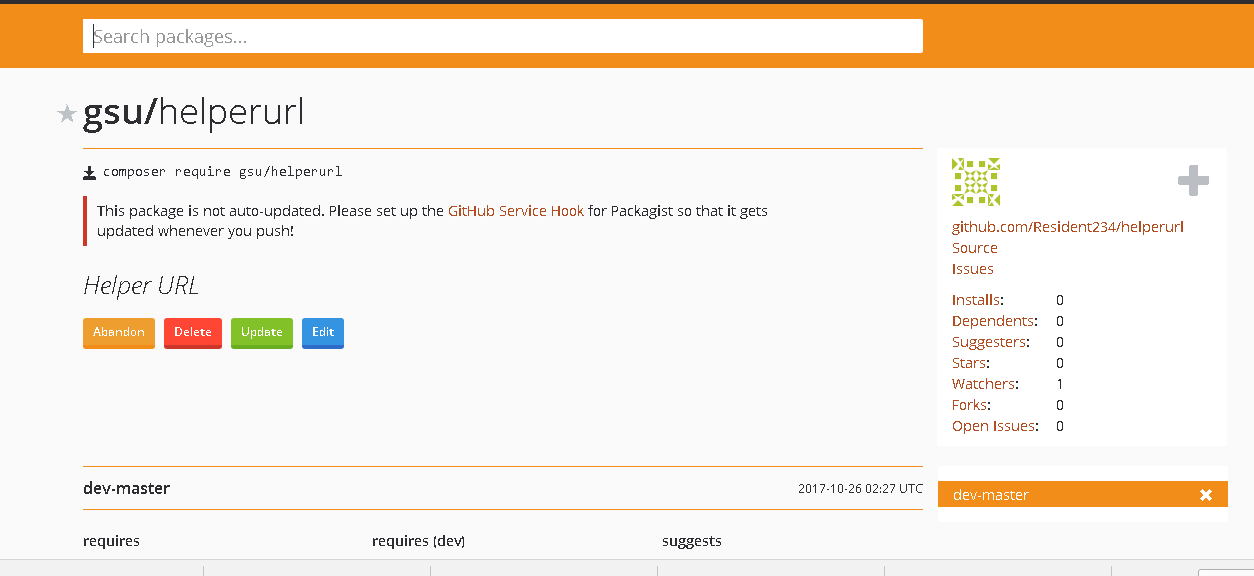
Checking the performance of the package
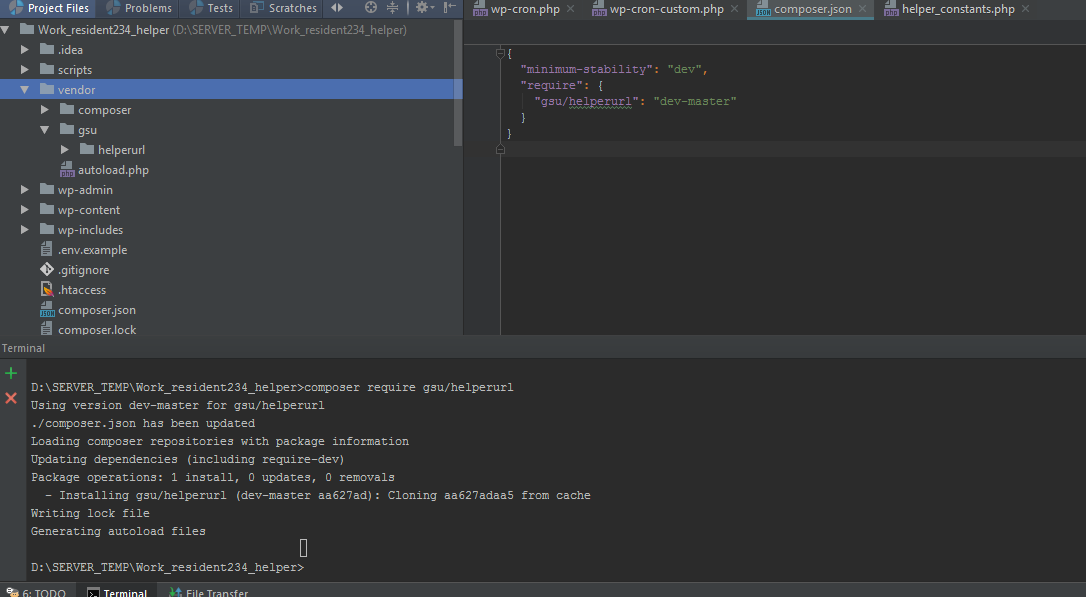
Package delivered, here is our class:
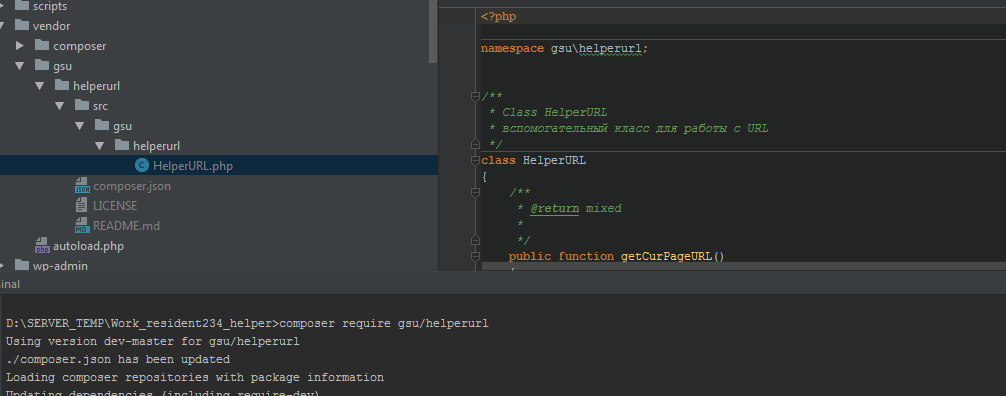
Call the method
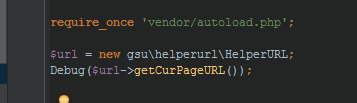
All OK.
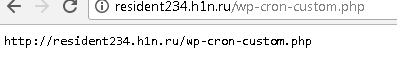
Composer and PhpStorm
Initialization
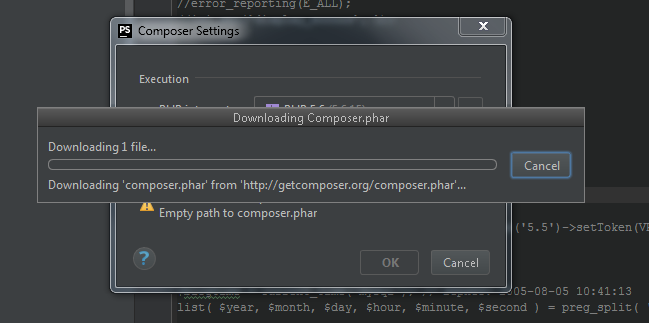
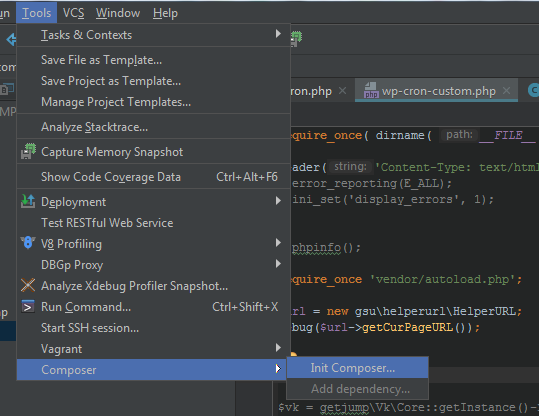
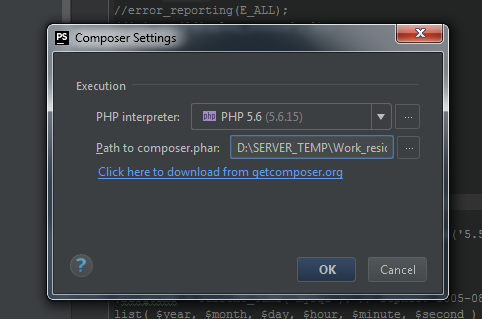

Configuring the ability to edit Composer packages
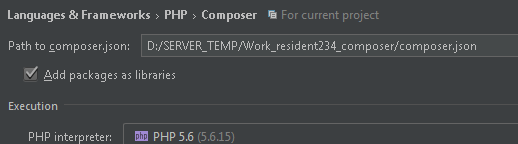
If the option is set, it will not be so easy to take and edit the files inside the vendor / * / *
Package installation
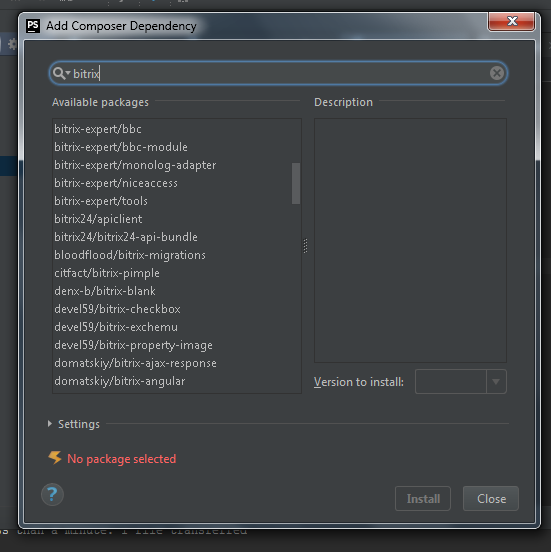
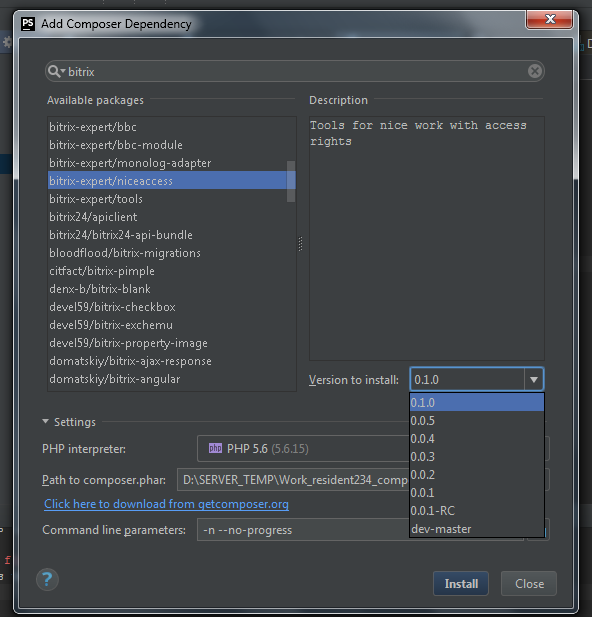

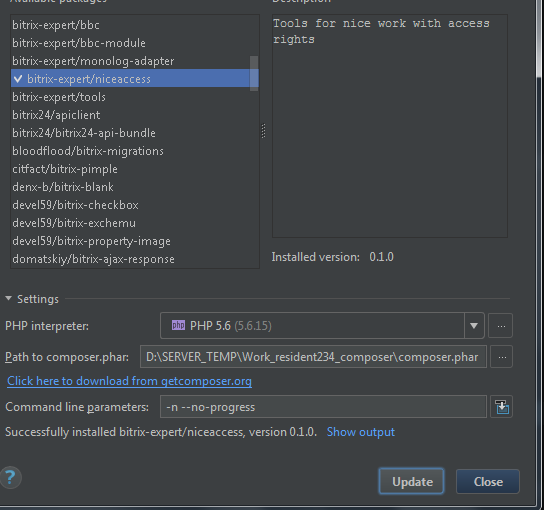
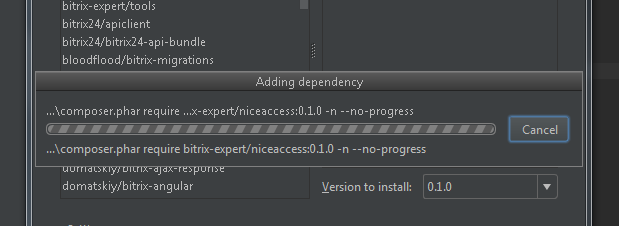
Nuances, subtleties, difficult situations
Error: Warning: The Complement in Composer.json. You may be getting outdated by dependencies. Run update to update them. Nothing to install or update
Solution: composer update - lock
Long update is performed when a large number of installed libraries
Composer checks all package dependencies, and if there are many packages, then this is a long time.
Solution: if you need to update only one library, then we specify it explicitly:
composer update package / nameYou can also add the parameter "--prefer-dist" (although, in theory, it should be enabled by default), then the composer will try to install the library from the zip archive, and not clone the repository.
The "****. Json" file could not be downloaded: failed request!
Composer is trying to pull a packet over HTTP, although it needs HTTPS
Solution: composer config --global repo.packagist composer
packagist.orgYour package is not available.
see for more details.
The package does not have a stable version, and installing the dev version is not allowed in the config.
Solution: either set the “minimum-stability” parameter: “dev” and “prefer-stable”: true to install stable versions as much as possible, or - if this is your own package - create a tag with the version (the sticker in the readme on the github should show version)
Development history and key changes
- the first release took place on March 1, 2012 and the whole 2012 instrument is actively developing
- January 2014 - PSR-4 based autoload implemented
- March 2016 - beta version (1.0.0-beta1) was released. The show --tree commands have been added to display the installed packages as a tree, why-not — shows why the package cannot be fixed, update --interactive - allows you to select which packages to update, as well as many other improvements and fixes.
- April 4, 2016 - the first stable release of Composer was introduced - 1.0.0
December 2014 - one of the key commits to the composer repository
github.com/composer/composer/commit/ac676f47f7bbc619678a29deae097b6b0710b799
The essence of the change - the garbage collector is disabled

habrastorage.org/getpro/habr/comment_images/db3/59a/972/db359a972df6730e52f292b5c52095b8.gifLinks
Offsite: getcomposer.org
Official package repository: packagist.org
Composer repository:
github.com/composer/composerGreat great tutorial on using Composer:
daylerees.com/composer-primerList of commands and a detailed example of the composer.json file:
composer.json.jolicode.com