There is a standard procedure for the latest versions of
Javascript and there is a whole committee behind it. In the article I will talk about who makes decisions on any new specification, what is the procedure for it and what's new in
ES2019 .
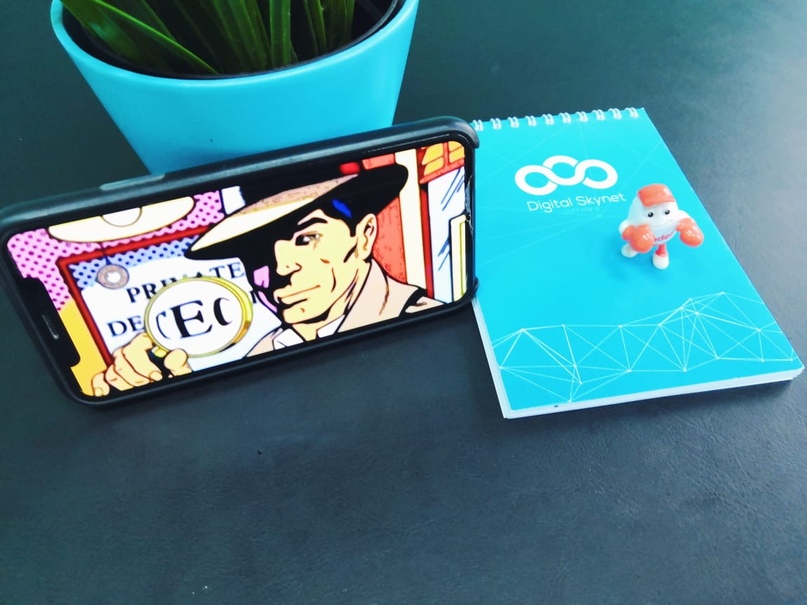
The language specification that governs JavaScript is called
ECMAScript . There is a group called Technical Committee
39 [TC39] , which reviews each specification before adoption.
Each change takes place in stages:
- Stage 0: Ideas
- Stage 1: Suggestions
- Stage 2: Drafts
- Stage 3: Candidates
- Stage 4: Completion / Approval
A function that reaches stage 4 is likely to be part of the language specification.
Consider what is added to the specification in accordance with ES2019.
Array.prototype. {Flat, flatMap}
Array.prototype.flat () allows you to recursively smooth arrays to a predetermined depth and return a new array.
Syntax:
Array.prototype.flat (depth)Depth is the default value of 1; use
Infinity to smooth out all nested arrays.
const numbers = [1, 2, [3, 4, [5, 6]]]; // Considers default depth of 1 numbers.flat(); > [1, 2, 3, 4, [5, 6]] // With depth of 2 numbers.flat(2); > [1, 2, 3, 4, 5, 6] // Executes two flat operations numbers.flat().flat(); > [1, 2, 3, 4, 5, 6] // Flattens recursively until the array contains no nested arrays numbers.flat(Infinity) > [1, 2, 3, 4, 5, 6]
Array.prototype.flatMap () displays each element using the display function and aligns the result to a new array. This is identical to the
map operation, followed by a
flat depth of 1.
Syntax:
Array.prototype.flatMap (callback)callback: the
function that produces the element of the new array.
const numbers = [1, 2, 3]; numbers.map(x => [x * 2]); > [[2], [4], [6]] numbers.flatMap(x => [x * 2]); > [2, 4, 6]
Object.fromEntries
Object.fromEntries does the opposite of Object.entries. It converts a list of key-value pairs to an object.
Syntax:
Object.fromEntries (iterable)iteration: Iteration as an
Array or
Map objects that implement the
protocol being iterated .
const records = [['name','Mathew'], ['age', 32]]; const obj = Object.fromEntries(records); > { name: 'Mathew', age: 32} Object.entries(obj); > [['name','Mathew'], ['age', 32]];
String.prototype. {TrimStart, trimEnd}
trimStart () removes spaces at the beginning of a line, and
trimEnd () removes spaces at the end of a line.
const greeting = ` Hello Javascript! `; greeting.length; > 19 greeting = greeting.trimStart(); > 'Hello Javascript! ' greeting.length; > 18 greeting = 'Hello World! '; greeting.length; > 15 greeting = greeting.trimEnd(); > 'Hello World!' greeting.length; > 12
Optional Catch Binding
Prior to the new specification, it was required to bind the exception variable to the
catch operator. ES2019 made it optional.
// Before try { ... } catch(error) { ... } // After try { ... } catch { ... }
This feature is useful if you want to completely ignore the error.
Best practice is to consider handling errors.There are cases when you know a possible error that may work when performing operations. And you can ignore catch handling.
JSON ECMAScript
Line terminator (U + 2028) and paragraph separator (U + 2029) characters are now allowed in string literals. Previously, they were treated as line terminators and resulted in
SyntaxError exceptions.
// Produces invalid string before ES2019 eval('"\u2028"'); // Valid in ES2019 eval('"\u2028"');
Well formed JSON.stringify
Instead of unpaired surrogate code points leading to single
UTF-16 code units, ES10 represents them using JSON escape sequences.
JSON.stringify('\uD800'); > '" "' JSON.stringify('\uD800'); > '"\\ud800"'
Function.prototype.toString
.toString () now returns exact snippets of source code text, including spaces and comments.
function /* a comment */ foo () {} // Previously: foo.toString(); > 'function foo() {}' ^ no comment ^ no space // Now: foo.toString(); > 'function /* comment */ foo () {}'
Symbol.prototype.description
A read-only property that returns an optional description of a
Symbol object:
Symbol('desc').toString(); > "Symbol(desc)" Symbol('desc').description; > "desc" Symbol('').description; > "" Symbol().description; > undefined
Conclusion
There are many other interesting things, such as static and private methods and fields in classes, Legacy RegEx, etc. It is important for the developer to monitor the emergence of new technologies, the improvement of old ones and to use it in work.
It will be useful to read previous versions:
ES2015 ES2016 ES2017 ES2018